Multi Select Box With Tree Structured Data Dropdown List
Dmitry Zhuravkov February 10, 2023
A user-friendly multi select JS library that allows the user to select single or multiple options from a tree-style hierarchical dropdown list.
Implementation
Import the Treeselect's JavaScript and Stylesheet
import Treeselect from '../dist/treeselect-js.mjs.js';
Create an empty DIV container to hold the Multi-Select dropdown box
Define your nested options as follows
const options = [ { name: 'JavaScript', value: 'JavaScript', children: [ { name: 'React', value: 'React', children: [ { name: 'React.js', value: 'React.js', children: [] }, { name: 'React Native', value: 'React Native', children: [] } ] }, { name: 'Vue', value: 'Vue', children: [] } ] }, { name: 'HTML', value: 'html', children: [ { name: 'HTML5', value: 'HTML5', children: [] }, { name: 'XML', value: 'XML', children: [] } ] } ]
Initialize the Treeselect and populate the Multi-Select dropdown box with the data you just created
const treeselect = new Treeselect({ parentHtmlContainer: document.querySelector('.example'), options: options, })
Available options to customize the Treeselect
const treeselect = new Treeselect({ // parent container element parentHtmlContainer: HTMLElement, // an array of option values value: [], // define your options here options: [], // keep the tree select always open alwaysOpen: true, // open all options to this level openLevel: 0, // append the Treeselect to the body appendToBody: false, // shows selected options as tags showTags: true, // text to show if you use 'showTags' tagsCountText: '{count} {tagsCountText}', // shows remove icon clearable: true, // is searchable? searchable: true, // placeholder text placeholder: 'Search...', // text to display when no results found emptyText: 'No results found...', // converts multi-select to the single value select isSingleSelect: false, // returns groups if they selected instead of separate ids isGroupedValue: false, // It is impossible to select groups. You can select only leaves. disabledBranchNode: false, // auto, top, bottom direction: 'auto', // shows count of children near the group's name. showCount: false, // group options grouped: true, // element that appends to the end of the dropdown list listSlotHtmlComponent: null, // is dropdown list disabled? disabled: false, // add the list as a static DOM element // this prop will be ignored if you use appendToBody. staticList: false, // id attribute for the accessibility. id: '', // { arrowUp, arrowDown, arrowRight, attention, clear, cross, check, partialCheck } // object contains all svg icons iconElements: {}, srcElement: null, // callback and functions inputCallback: undefined, openCallback: undefined, closeCallback: undefined, nameChangeCallback: undefined, mount: () => void, updateValue: (newValue: ValueOptionType[]) => void, destroy: () => void, focus: () => void, toggleOpenClose: () => void, })
Trigger a function every time an option has been selected
treeselect.srcElement.addEventListener('input', (e) => { console.log(e.detail) })
API methods
// update options treeselect.updateValue(Array[String]); // remount and update settings treeselect.mount(); // toggle between open & close treeselect.toggleOpenClose(); // set focus on treeselect input without open/close state changes. treeselect.focus(); // destroy the instance treeselect.destroy();
Next: How to Train ChatGPT With Your Own Website Data
also view other related posts,
Zoise Angular Business Website Template
Zoise is a simple and responsive website template for businesses with dark and light modes, suitable for business professionals and designers.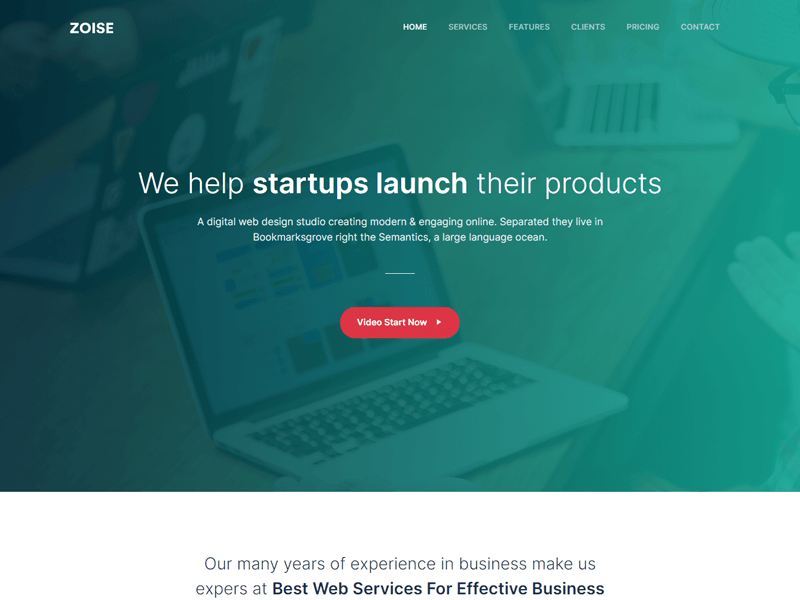
Tailbreeze Admin Dashboard Template
Tailbreeze: Responsive admin template with sophisticated design, built using TailwindCSS, offering application views, widgets, and components.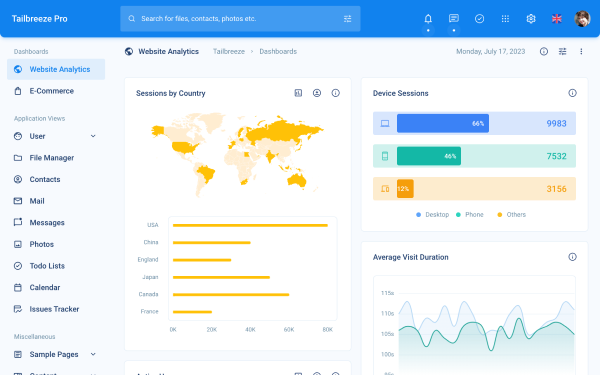